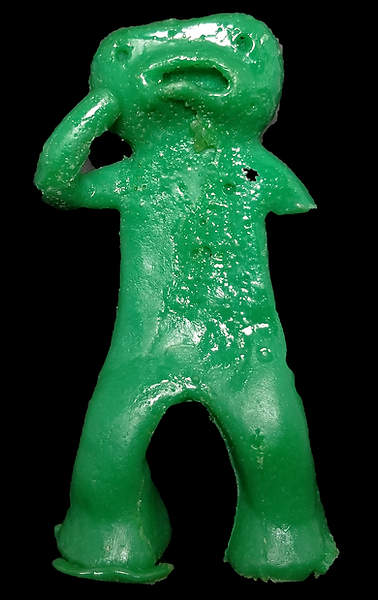
Justin W.H. Yuen

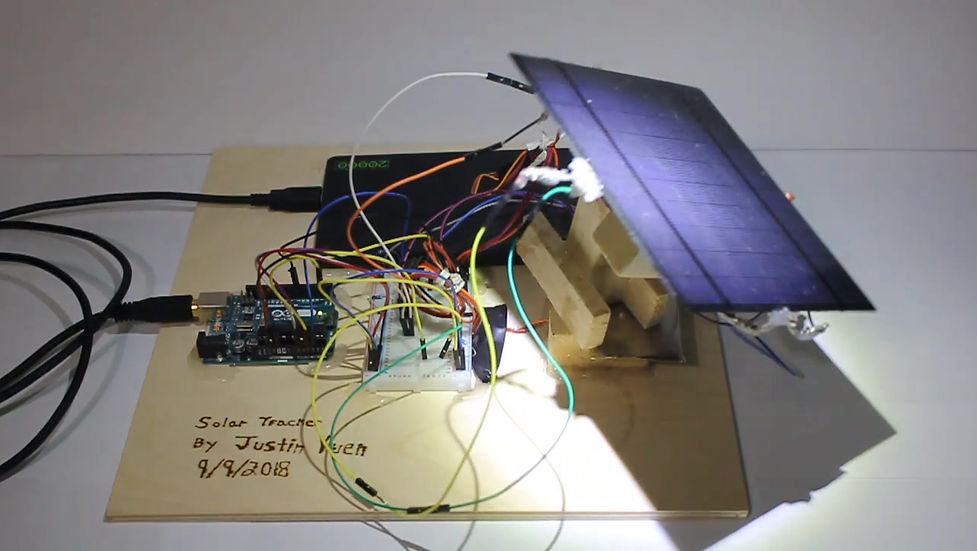
This was my first project where I designed and built an idea from the ground up. It was also my first time working with an Arduino and solar panel.
Goal
Program an Arduino to find and face the brightest source of light
Status
Complete
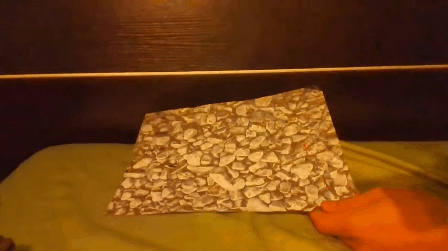
What inspired me to start this project was seeing new ships and boats taking advantage of solar panels to power their motors. To visualize my ideas, I learned how to make a phone app with Unity that would allow me to see my 3D CAD file using augmented reality.


I used affordable and lightweight balsa wood from local arts and crafts stores to build the tower of the solar panel.
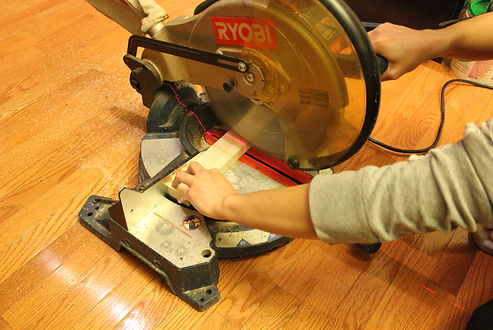

Although wood cuts easily, bringing the saw down slowly gives a cleaner cut.
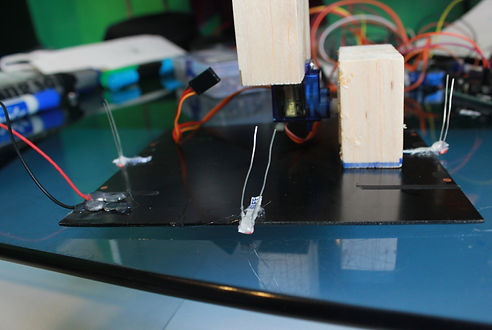
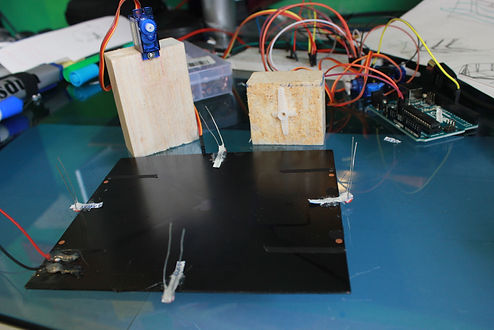
Blocks of balsa to form the tower and the solar panel (black square).
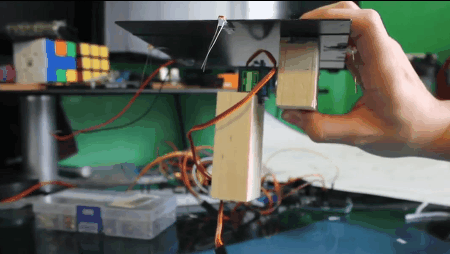
How the servo motor would rotate the solar panel and its range of motion.
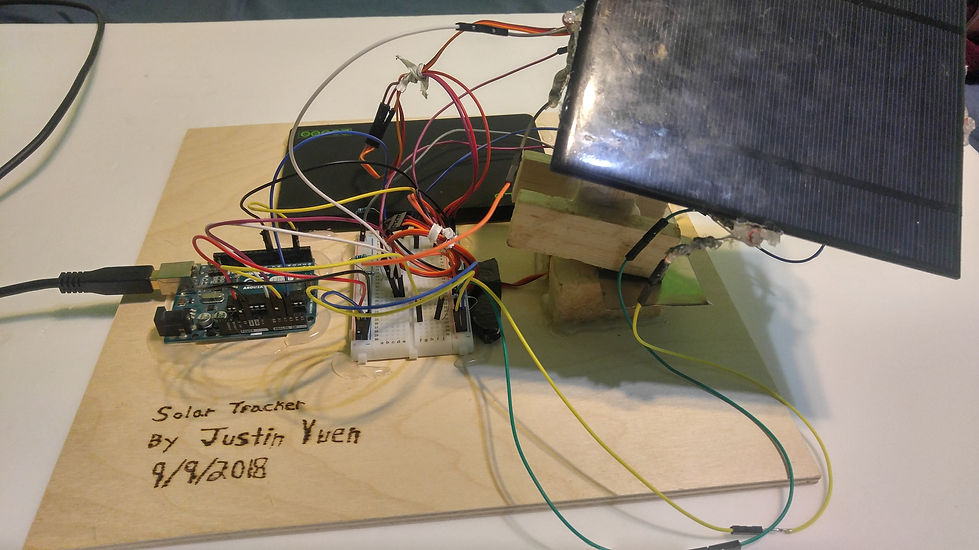
Full assembly of the Arduino-controlled solar panel.
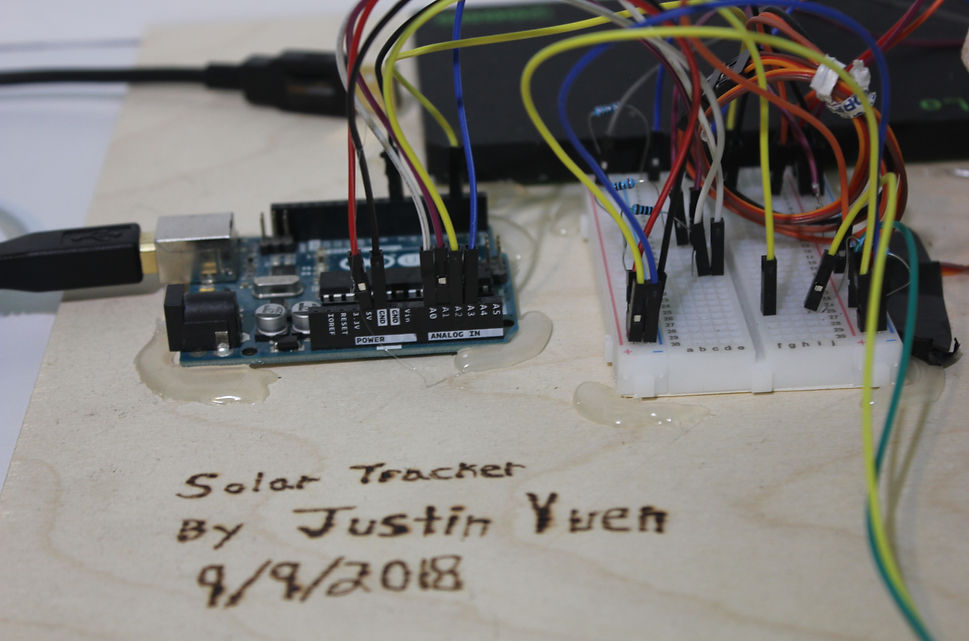
My Arduino Code
Feel free to ask me to clarify any lines in the code. I would be glad to help!
//adding LDRs
const int ldrPinL = A0; // white for left
const int ldrPinR = A1; //purple for right
const int ldrPinF = A2; //yellow for front
const int ldrPinB = A3; //blue for back
// one end of ldr to power, other to ground. must add resistor or value too low
//photoresistors used are 5505 - resistance ranges between 2-6kOhm
//adding servos
#include <Servo.h>
Servo servot; //top servo
Servo servob; //bottom servo
int angle; //angle is gonna represent the angle of the servos
void setup() {
Serial.begin(9600);
pinMode(ldrPinL, INPUT);
pinMode(ldrPinR, INPUT);
pinMode(ldrPinF, INPUT);
pinMode(ldrPinB, INPUT);
servot.write(110); //set initial angle to 150
servot.attach(2); //top servo connected to port 2
servob.write(110); //set initial angle to 150
servob.attach(7); //top servo connected to port 7
}
void loop() {
int ldrStatusL = analogRead(ldrPinL);
int ldrStatusR = analogRead(ldrPinR);
int ldrStatusF = analogRead(ldrPinF);
int ldrStatusB = analogRead(ldrPinB);
int differenceLR = (ldrStatusL-ldrStatusR); //difference between left and right LDRs
int differenceFB = (ldrStatusF-ldrStatusB); //difference between front and back LDRs
int initialangle = 150; //initial servo position
/*
Serial.println("left ldr is");
Serial.println(ldrStatusL);
Serial.println("right ldr is");
Serial.println(ldrStatusR);
Serial.println("front ldr is");
Serial.println(ldrStatusF);
Serial.println("back ldr is");
Serial.println(ldrStatusB);
*/
//Serial.println(differenceLR);
Serial.println("top");
Serial.println(servot.read());
//Serial.println(differenceFB);
Serial.println("bottom");
Serial.println(servob.read());
if (differenceLR>=40) {
//Serial.println("LR NOT CENTERED");
if (servob.read()<180) {
servob.write(servob.read()-10);
}
}
else if (differenceLR<=-40) {
//Serial.println("LR NOT CENTERED");
servob.write(servob.read()+10);
}
else{
// Serial.println("LR is centered!");
}
if (differenceFB>=40){
//Serial.println("FB NOT CENTERED");
if (servot.read()<180) {
servot.write(servot.read()+10);
}
}
else if (differenceFB<=-40) {
//Serial.println("FB NOT CENTERED");
servot.write(servot.read()-10);
}
else{
//Serial.println("FB is centered!");
}
delay(1000);
}